# Simple Recyclerview Android Example in Kotlin
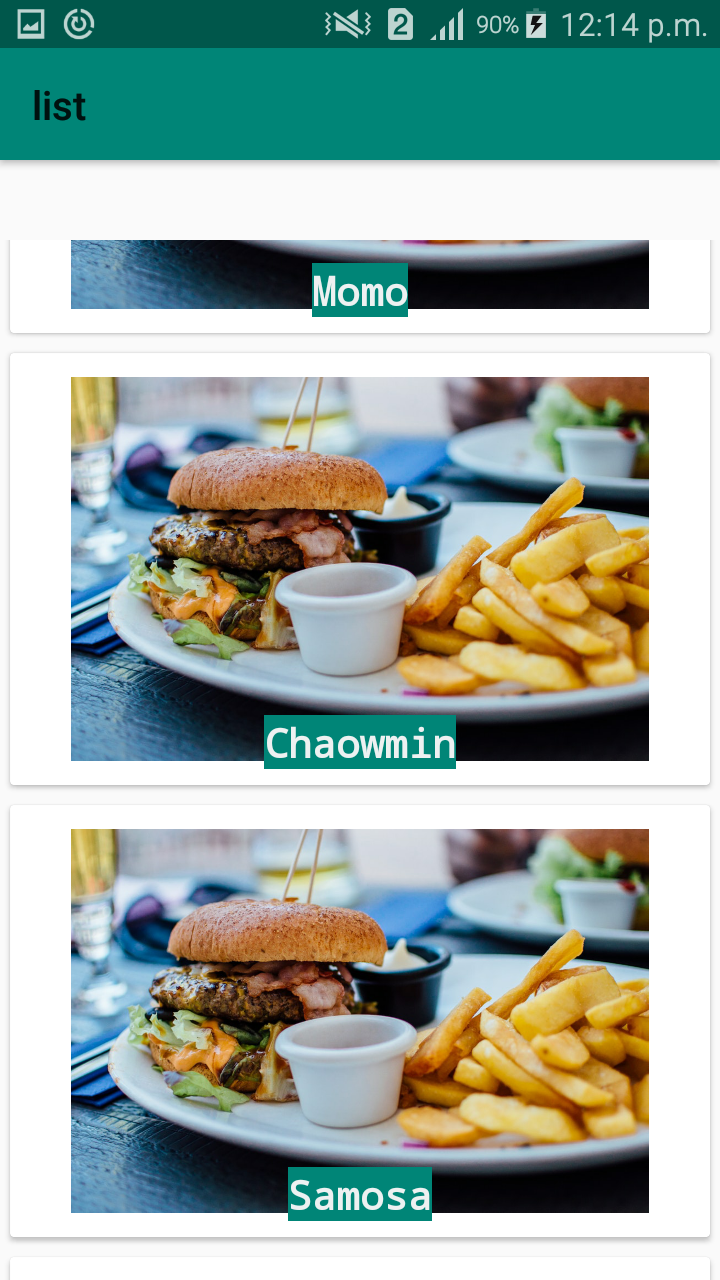
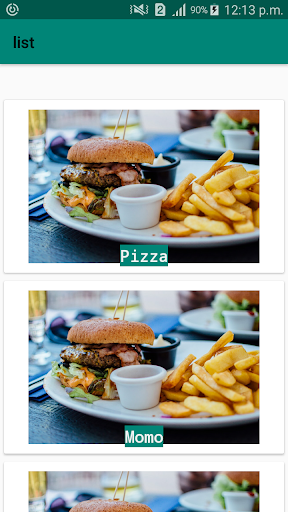
If your app needs to display a scrolling list of elements based on large data sets (or data that frequently changes), you should use RecyclerView as described on this page.
# Complete Code
# UI Design
- Design for each row of the list:
It consists of the Linear Layout with the Card View . Each row consist of a
ImageView
andTextView
food_list_row.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="8dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="fill_parent"
android:layout_height="200dp"
android:padding="4dp"
android:contentDescription="Image"
android:src="@drawable/food"
/>
<TextView
android:id="@+id/foodName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Food Name"
android:textAppearance="@style/Base.TextAppearance.AppCompat.Small"
android:textColor="#EDF1F1"
android:textStyle="bold"
android:layout_alignBottom="@id/imageView"
android:layout_centerHorizontal="true"
android:background="@color/colorPrimary"
android:capitalize="characters" app:fontFamily="monospace"
android:layout_marginTop="40dp" android:layout_marginLeft="20dp" android:layout_marginStart="20dp"
android:layout_marginRight="20dp" android:layout_marginEnd="20dp"
android:textSize="20dp"/>
</RelativeLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
- Include RecyclerView in the Main XMl file:
In activity_main.xml
<android.support.v7.widget.RecyclerView
android:id="@+id/Food"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginTop="40dp"
tools:layout_editor_absoluteX="0dp" />
1
2
3
4
5
6
7
2
3
4
5
6
7
# Add Necessary Library
build.gradle
dependencies {
......
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
}
1
2
3
4
5
6
2
3
4
5
6
# Create Model Representing Data for each row
Food_Model.kt
data class Food_Model(
var foodID: String ="",
var foodName: String = ""
)
1
2
3
4
5
6
2
3
4
5
6
# Create Adapter for RecyclerView
An adapter acts like a bridge between a data source and the user interface. It reads data from various data sources, coverts it into View objects and provide it to the linked Adapter view to create UI components.
It needs folowing dependencies
- Layout File :
food_list_row.xml
- Model :
Food_Model.kt
import android.content.Context
import android.support.v7.widget.RecyclerView
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import kotlinx.android.synthetic.main.food_list_row.view.*
class Food_Adapter(context: Context,private val Food: ArrayList<Food_Model>
) : RecyclerView.Adapter<Food_Adapter.ViewHodler>() {
private val mContext: Context
init {
mContext = context
}
override fun onCreateViewHolder(parent: ViewGroup, p1: Int): Food_Adapter.ViewHodler {
val food_row = LayoutInflater.from(mContext).inflate(R.layout.food_list_row,parent,false)
return ViewHodler(food_row)
}
override fun getItemCount(): Int {
return Food.size
}
override fun onBindViewHolder(holder: Food_Adapter.ViewHodler, position: Int) {
val model = Food[position]
holder.foodName!!.text = model.foodName
}
inner class ViewHodler(v : View) : RecyclerView.ViewHolder(v) {
val foodName = v.foodName
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
# Update Main Activity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
food_listview = findViewById<View>(R.id.Food) as RecyclerView
food_listview.layoutManager = LinearLayoutManager(this)
adapter = Food_Adapter(this@MainActivity, foodList)
food_listview.adapter = adapter// set adapter
prepareFoodData()
}
companion object {
lateinit var food_listview: RecyclerView
var foodList = ArrayList<Food_Model>()
private var adapter: Food_Adapter? = null
}
private fun prepareFoodData() {
foodList.add( Food_Model("1", "Pizza"))
foodList.add( Food_Model("2", "Momo"))
foodList.add( Food_Model("3", "Chaowmin"))
foodList.add( Food_Model("4", "Samosa"))
foodList.add( Food_Model("5", "Aalu Stick"))
foodList.add( Food_Model("6", "Burger"))
adapter!!.notifyDataSetChanged()
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34